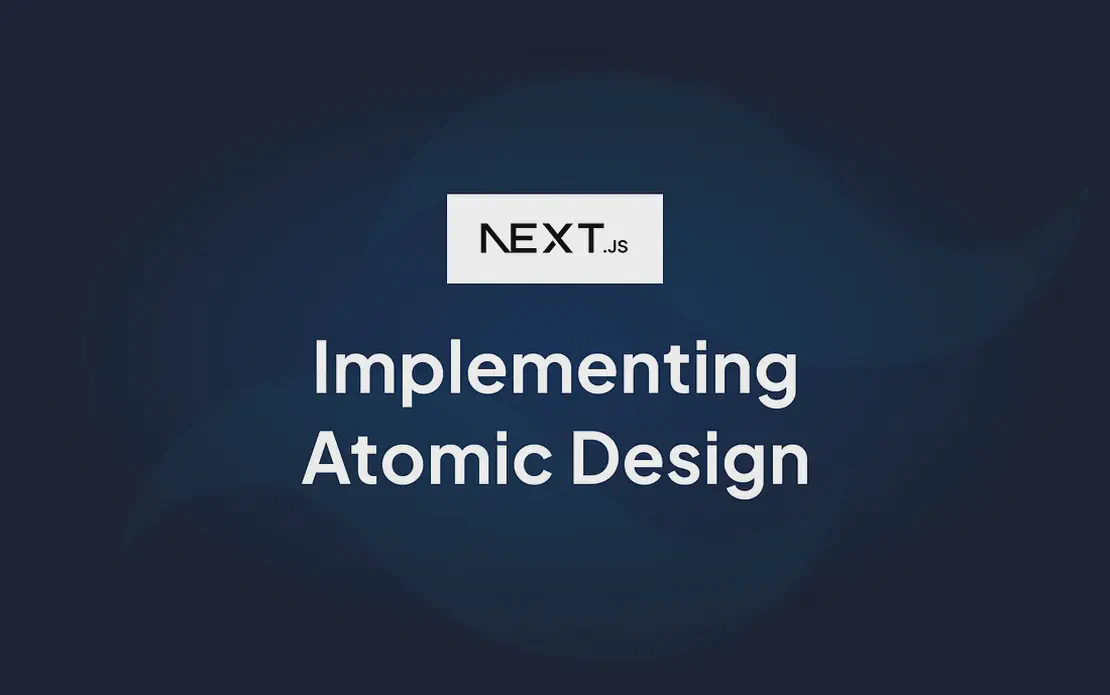
Atomic Design with Next.js: A Step-by-Step Guide
- Beta Priyoko
- Web development , Ui ux design , Web development , Next.js
- September 26, 2024
Introduction: What is Atomic Design?
Atomic Design is a methodology for creating design systems with a clear, hierarchical structure. It breaks down UI components into five distinct levels: Atoms, Molecules, Organisms, Templates, and Pages. This approach helps create consistent and maintainable UI components, making it especially useful when building modern web applications like those developed with Next.js.
Why Use Atomic Design in Next.js?
- Scalability: Easily manage large codebases with reusable components.
- Consistency: Design consistency across the application with repeatable patterns.
- Maintainability: Easier updates and bug fixes by focusing on individual components.
- Collaboration: Simplifies communication between developers and designers.
Atomic Design Principles
Atomic Design breaks down UI elements into five levels:
- Atoms: Basic building blocks (e.g., buttons, inputs, labels).
- Molecules: Combinations of atoms working together (e.g., a search bar).
- Organisms: Complex structures composed of molecules and/or atoms (e.g., a navbar).
- Templates: Layout structures with placeholders for content (e.g., a page layout).
- Pages: Real implementations of templates with actual content.
Implementing Atomic Design in Next.js
1. Setting Up Your Next.js Project
First, create a new Next.js project if you haven’t already:
npx create-next-app@latest nextjs-atomic-design
cd nextjs-atomic-design
npm install
2. Structuring the Project
Create a folder structure to reflect the Atomic Design methodology:
src/
│
├── components/
│ ├── atoms/
│ │ ├── Button.tsx
│ │ ├── Input.tsx
│ │ └── Label.tsx
│ │
│ ├── molecules/
│ │ ├── SearchBar.tsx
│ │ └── FormGroup.tsx
│ │
│ ├── organisms/
│ │ ├── Navbar.tsx
│ │ └── HeroSection.tsx
│ │
│ ├── templates/
│ │ └── MainLayout.tsx
│ │
│ └── pages/
│ └── HomePage.tsx
│
├── pages/
│ ├── index.tsx
│ └── _app.tsx
│
└── styles/
└── globals.css
3. Building Components Step-by-Step
Atoms: Basic Building Blocks
Button Component (atoms/Button.tsx
):
// src/components/atoms/Button.tsx
import React from 'react';
type ButtonProps = {
label: string;
onClick: () => void;
};
const Button: React.FC<ButtonProps> = ({ label, onClick }) => {
return (
<button
className="px-4 py-2 bg-blue-500 text-white rounded hover:bg-blue-600"
onClick={onClick}
>
{label}
</button>
);
};
export default Button;
Input Component (atoms/Input.tsx
):
// src/components/atoms/Input.tsx
import React from 'react';
type InputProps = {
placeholder: string;
value: string;
onChange: (e: React.ChangeEvent<HTMLInputElement>) => void;
};
const Input: React.FC<InputProps> = ({ placeholder, value, onChange }) => {
return (
<input
type="text"
placeholder={placeholder}
value={value}
onChange={onChange}
className="border p-2 rounded"
/>
);
};
export default Input;
Molecules: Combining Atoms
SearchBar Component (molecules/SearchBar.tsx
):
// src/components/molecules/SearchBar.tsx
import React, { useState } from 'react';
import Input from '../atoms/Input';
import Button from '../atoms/Button';
const SearchBar: React.FC = () => {
const [query, setQuery] = useState('');
const handleSearch = () => {
alert(`Searching for: ${query}`);
};
return (
<div className="flex items-center space-x-2">
<Input
placeholder="Search..."
value={query}
onChange={(e) => setQuery(e.target.value)}
/>
<Button label="Search" onClick={handleSearch} />
</div>
);
};
export default SearchBar;
Organisms: Combining Molecules
Navbar Component (organisms/Navbar.tsx
):
// src/components/organisms/Navbar.tsx
import React from 'react';
import SearchBar from '../molecules/SearchBar';
const Navbar: React.FC = () => {
return (
<nav className="p-4 bg-gray-800 text-white flex justify-between">
<div className="text-xl font-bold">MyApp</div>
<SearchBar />
</nav>
);
};
export default Navbar;
Templates: Creating Layout Structures
MainLayout Component (templates/MainLayout.tsx
):
// src/components/templates/MainLayout.tsx
import React from 'react';
import Navbar from '../organisms/Navbar';
type MainLayoutProps = {
children: React.ReactNode;
};
const MainLayout: React.FC<MainLayoutProps> = ({ children }) => {
return (
<div>
<Navbar />
<main className="p-6">{children}</main>
</div>
);
};
export default MainLayout;
Pages: Building Real Implementations
HomePage Component (pages/HomePage.tsx
):
// src/components/pages/HomePage.tsx
import React from 'react';
import MainLayout from '../templates/MainLayout';
const HomePage: React.FC = () => {
return (
<MainLayout>
<h1 className="text-2xl font-bold">Welcome to the Home Page</h1>
<p className="mt-4">This is a simple implementation of Atomic Design with Next.js.</p>
</MainLayout>
);
};
export default HomePage;
Using the HomePage in Next.js (pages/index.tsx
):
// pages/index.tsx
import HomePage from '@/components/pages/HomePage';
export default function Index() {
return <HomePage />;
}
Conclusion
Implementing Atomic Design with Next.js helps create a maintainable and scalable application. By breaking down the UI into reusable components, you can streamline the development process, keep your code organized, and enhance collaboration between designers and developers. Try incorporating this design pattern into your Next.js projects to experience the benefits firsthand.