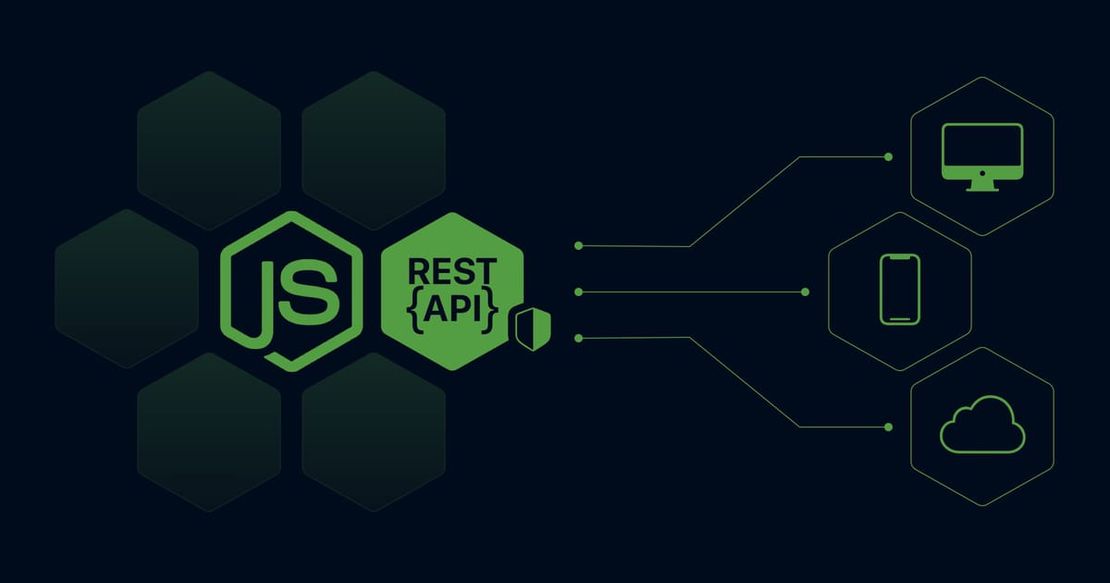
Essential Considerations and Best Practices for Developing an Express.js Backend API
- Beta Priyoko
- Web development , Backend development , Java script frameworks , Apis
- September 27, 2024
Introduction
In today’s digital landscape, creating a robust backend API is essential for the success of web applications. Express.js, a minimal and flexible Node.js web application framework, has become the go-to choice for developers looking to build APIs efficiently. It offers a simple structure and powerful features, making it an ideal choice for both beginners and experienced developers.
This blog post explores essential considerations and best practices for developing an Express.js backend API. We’ll cover everything from setting up your environment and designing your API to implementing security measures and optimizing performance. By following these guidelines, you can create a scalable, maintainable, and secure backend service that meets your application’s needs.
Setting Up the Environment
Node.js and Express Installation
To get started, ensure that you have Node.js installed on your machine. You can download it from Node.js official website. Once installed, create a new directory for your project and initialize a new Node.js application:
mkdir my-express-api
cd my-express-api
npm init -y
Next, install Express and other necessary packages:
npm install express dotenv mongoose
Project Structure
A well-organized project structure is crucial for maintainability. Here’s a recommended folder structure:
my-express-api/
├── src/
│ ├── config/ # Configuration files
│ ├── controllers/ # Route handlers
│ ├── middleware/ # Custom middleware
│ ├── models/ # Database models
│ ├── routes/ # API routes
│ ├── utils/ # Utility functions
│ └── app.js # Main application file
├── .env # Environment variables
├── package.json # Project metadata
└── README.md # Documentation
Environment Configuration
Using environment variables helps manage sensitive information securely. Create a .env
file in the root of your project:
PORT=3000
MONGO_URI=mongodb://localhost:27017/mydatabase
JWT_SECRET=mysecretkey
Load the environment variables in your application:
require('dotenv').config();
Designing the API
RESTful API Design Principles
Following RESTful principles ensures your API is predictable and intuitive. Use nouns for resource names and HTTP methods to define actions. For example:
GET /api/users
- Retrieve a list of usersPOST /api/users
- Create a new userGET /api/users/:id
- Retrieve a specific userPUT /api/users/:id
- Update a specific userDELETE /api/users/:id
- Delete a specific user
Versioning Your API
API versioning is critical for managing changes without disrupting existing clients. Include the version in your URL:
app.use('/api/v1', userRoutes);
Handling Different HTTP Methods
Use appropriate HTTP methods to represent actions on resources. For instance, use GET
for fetching data, POST
for creating resources, PUT
for updating, and DELETE
for removing.
Middleware Management
Built-in vs. Custom Middleware
Express provides built-in middleware for parsing request bodies and handling CORS. You can also create custom middleware for specific tasks. Here’s an example of a simple logging middleware:
const logger = (req, res, next) => {
console.log(`${req.method} ${req.url}`);
next();
};
app.use(logger);
Error Handling Middleware
Centralized error handling is essential for managing unexpected issues. Define an error handling middleware at the end of your middleware stack:
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).json({ message: 'Internal Server Error' });
});
Request Validation Middleware
Use libraries like express-validator
or Joi
to validate incoming requests. Here’s an example using express-validator
:
const { body, validationResult } = require('express-validator');
app.post('/api/users',
body('name').isString().isLength({ min: 3 }),
(req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
// Continue with user creation...
}
);
Security Best Practices
Securing Endpoints with JWT
JSON Web Tokens (JWT) are widely used for securing routes. Use middleware to verify tokens on protected routes:
const jwt = require('jsonwebtoken');
const authenticateJWT = (req, res, next) => {
const token = req.headers['authorization'];
if (token) {
jwt.verify(token, process.env.JWT_SECRET, (err, user) => {
if (err) {
return res.sendStatus(403);
}
req.user = user;
next();
});
} else {
res.sendStatus(401);
}
};
app.get('/api/users', authenticateJWT, (req, res) => {
// Fetch users...
});
Input Sanitization and Validation
Always sanitize and validate user input to prevent SQL Injection and XSS attacks. Use libraries like express-validator
to handle this.
Rate Limiting and DDOS Protection
Implement rate limiting to prevent abuse. The express-rate-limit
package can help you easily enforce this:
const rateLimit = require('express-rate-limit');
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100 // Limit each IP to 100 requests per windowMs
});
app.use(limiter);
Securing HTTP Headers with Helmet
Use the helmet
middleware to set various HTTP headers for improved security:
const helmet = require('helmet');
app.use(helmet());
Performance Optimization
Caching Strategies
Implement caching strategies to reduce server load. Use Redis for caching frequently accessed data. Here’s a simple example of caching a user list:
const redis = require('redis');
const client = redis.createClient();
app.get('/api/users', (req, res) => {
client.get('users', (err, users) => {
if (users) {
return res.json(JSON.parse(users));
} else {
// Fetch from database, then cache it
User.find({}, (err, users) => {
client.setex('users', 3600, JSON.stringify(users)); // Cache for 1 hour
res.json(users);
});
}
});
});
Using Asynchronous Programming
Leverage async/await
to keep your code clean and non-blocking:
app.get('/api/users', async (req, res) => {
try {
const users = await User.find({});
res.json(users);
} catch (error) {
res.status(500).json({ message: 'Error fetching users' });
}
});
Compression with Gzip
Use the compression
middleware to compress response bodies, which can significantly reduce the size of data transferred:
const compression = require('compression');
app.use(compression());
Database Integration
Choosing the Right Database
Selecting the right database is crucial for your application’s performance. For document-based data, MongoDB is a popular choice. For relational data, consider PostgreSQL or MySQL.
Connecting with Mongoose or Sequelize
Use Mongoose for MongoDB or Sequelize for SQL databases. Here’s an example of connecting to MongoDB with Mongoose:
const mongoose = require('mongoose');
mongoose.connect(process.env.MONGO_URI, { useNewUrlParser: true, useUnifiedTopology: true })
.then(() => console.log('MongoDB connected'))
.catch(err => console.error('MongoDB connection error:', err));
Handling Transactions and Data Validation
Ensure data integrity with transactions (for SQL) or validation (for NoSQL). Mongoose provides built-in validation, while Sequelize supports transactions.
Error Handling and Logging
Centralized Error Handling
Create a centralized error handling middleware to catch and log errors globally, which can improve your debugging process.
Logging with Winston or Morgan
Integrate logging tools like Winston or Morgan to track application events and errors. Here’s a basic example using Morgan:
const morgan = require('morgan');
app.use(morgan('combined'));
Monitoring with Tools like Sentry
Consider using Sentry or similar tools to monitor application performance and track errors in real time.
Testing and Validation
Unit Testing with Jest
Unit tests ensure that individual functions work as expected. Here’s a simple test case using Jest:
test('adds 1 + 2 to equal 3', () => {
expect(1 + 2).toBe(3);
});
Integration Testing with Supertest
Integration tests verify that your API endpoints function correctly. Supertest can help you test your API easily:
const request = require('supertest');
const app = require('../src/app');
describe('GET /api/users', () => {
it('responds with json', async () => {
const response = await request(app).get('/api/users');
expect(response.status).toBe(200);
expect(response.body).toBeInstanceOf(Array);
});
});
Mocking Databases and Dependencies
Use mocking libraries
like Sinon or Jest’s mocking features to isolate tests from actual databases.
Documentation and Maintenance
API Documentation with Swagger
Using tools like Swagger or Postman can help document your API. This makes it easier for other developers to understand and use your endpoints.
Version Control with Git
Implement a version control system (like Git) to manage changes and collaborate effectively with your team.
Regular Maintenance and Updates
Regularly update dependencies and review your codebase for improvements. Schedule maintenance tasks to address technical debt.
Conclusion
Building a robust Express.js backend API requires careful planning and adherence to best practices. By following the guidelines outlined in this post, you can create a scalable, secure, and maintainable API that meets your application’s needs. Remember to continually evaluate and improve your API as technology and user requirements evolve. Happy coding!