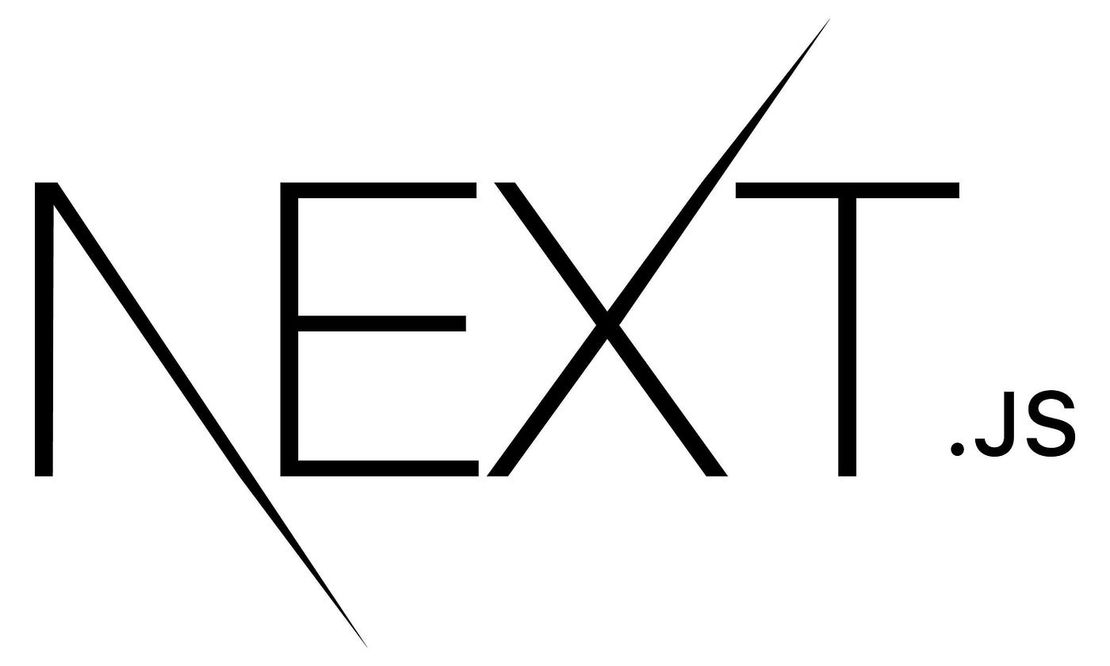
Essential Considerations in Frontend Development Using Next.js
- Beta Priyoko
- Web development , Java script frameworks
- October 5, 2024
Introduction:
Next.js is a powerful React framework that enables developers to build fast and scalable web applications. As you embark on your frontend development journey with Next.js, there are several essential considerations to keep in mind to optimize your application’s performance, maintainability, and user experience.
1. Understanding the Next.js Architecture
Next.js operates on a hybrid model, allowing for both static and server-side rendering (SSR). This architecture provides significant flexibility in how you serve content to users:
Static Generation (SSG): Pre-render pages at build time for faster load times. Ideal for content that doesn’t change often.
export async function getStaticProps() { const data = await fetchData(); return { props: { data }, // Will be passed to the page component as props }; }
Server-side Rendering (SSR): Generate pages on each request. This is suitable for dynamic content that requires fresh data.
export async function getServerSideProps() { const data = await fetchData(); return { props: { data }, }; }
Best Practice
Choose the rendering method based on the specific needs of your application. Consider using SSG for public pages and SSR for user-specific or frequently updated content.
2. Routing and File Structure
Next.js uses a file-based routing system, which simplifies navigation within your application. Understanding how to structure your files can improve organization and scalability.
Best Practice
- Organize your pages in the
pages
directory. Each.js
or.tsx
file corresponds to a route. - Utilize dynamic routes (e.g.,
[id].js
) for user profiles or product details. - Create nested folders for complex structures (e.g.,
/blog/[slug].js
for blog posts).
Example
/pages
/about.js
/blog
/[slug].js
/products
/[id].js
3. API Routes
Next.js supports API routes, allowing you to build serverless functions directly within your application. This is useful for handling backend logic without needing a separate server.
Best Practice
- Keep your API routes organized in the
pages/api
directory. - Use them for small backend tasks like form submissions or data fetching from external APIs.
// pages/api/hello.js export default function handler(req, res) { res.status(200).json({ message: 'Hello World' }); }
- Ensure proper error handling and response formatting.
4. State Management
Managing state in a Next.js application can be achieved through various libraries, such as React’s built-in useState
and useReducer
, or more complex state management tools like Redux or Zustand.
Best Practice
- For simpler state needs, start with React’s context and hooks.
- For larger applications, consider Redux or Zustand for better scalability and maintainability.
5. Styling and Theming
Next.js supports various styling solutions, including CSS Modules, styled-components, and Tailwind CSS. Choose a method that suits your project requirements and team preferences.
Best Practice
- Use CSS Modules for local scoping and avoid style conflicts.
- Tailwind CSS offers utility-first styling, promoting a clean and responsive design.
<div className="bg-blue-500 text-white p-4"> Hello, World! </div>
- Create a consistent theme using global styles and a theme provider for better maintainability.
6. Image Optimization
Next.js provides an <Image>
component that automatically optimizes images. This can lead to significant improvements in performance and loading times.
Best Practice
- Always use the Next.js
<Image>
component for images.import Image from 'next/image'; const MyImage = () => ( <Image src="/path/to/image.jpg" alt="Description" width={500} height={300} /> );
- Leverage lazy loading and specify width and height to avoid layout shifts.
- Optimize images at build time for different screen sizes.
7. Performance Optimization
Next.js includes several features that help improve application performance:
- Automatic Code Splitting: Only loads the necessary JavaScript for each page.
- Prefetching: Automatically prefetches linked pages for faster navigation.
Best Practice
- Monitor performance using tools like Google Lighthouse.
- Regularly analyze bundle sizes and optimize imports to keep them minimal.
- Enable HTTP/2 and leverage caching strategies for better performance.
Performance Benchmark Example
Using SSG, pages can load in under 500ms, while SSR might take 1-2 seconds depending on server response time.
8. Accessibility and SEO
Building accessible and SEO-friendly applications is crucial for user experience and reach.
Best Practice
- Use semantic HTML elements to improve accessibility.
- Ensure all images have alt text and consider ARIA attributes for assistive technologies.
- Leverage Next.js’s built-in SEO capabilities using the
next/head
component for meta tags and titles.import Head from 'next/head'; const MyPage = () => ( <Head> <title>My Page Title</title> <meta name="description" content="My page description." /> </Head> );
9. Testing and Quality Assurance
Implementing a robust testing strategy is vital for maintaining code quality and preventing bugs.
Best Practice
- Utilize tools like Jest and React Testing Library for unit and integration testing.
- Consider end-to-end testing with tools like Cypress to ensure a smooth user experience.
10. Deployment and Hosting
Next.js applications can be deployed to various platforms, including Vercel (the creators of Next.js), AWS, and Netlify.
Best Practice
- Choose a hosting provider that supports Next.js’s features like SSR and API routes.
- Implement continuous deployment strategies to streamline updates and improvements.
Common Pitfalls to Avoid
- Using SSR for pages that do not need real-time data, leading to unnecessary server load.
- Neglecting SEO practices, which can affect your application’s discoverability.
- Overcomplicating state management, leading to unnecessary complexity.
Further Resources
Conclusion
By considering these essential aspects of frontend development using Next.js, you can create robust, scalable, and user-friendly applications. Whether you’re building a simple blog or a complex web app, adhering to best practices will help ensure the success of your project.
What are your favorite features of Next.js? Share your experiences or questions in the comments below!