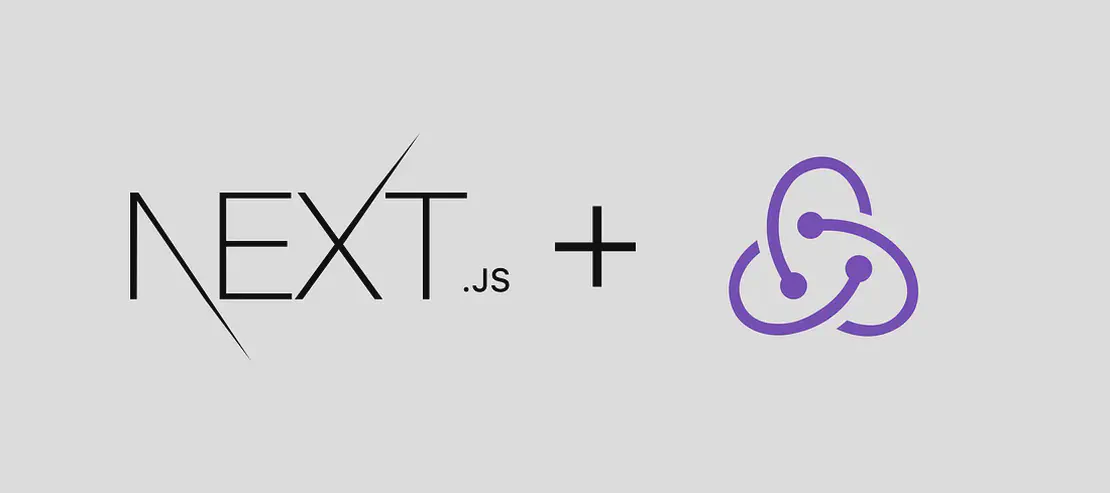
Optimizing Redux Toolkit Query for Large-Scale Next.js Applications
- Beta Priyoko
- Next.js , Redux toolkit , Web development , API management
- September 19, 2024
Introduction:
As your Next.js application grows, managing API requests efficiently becomes crucial. Using Redux Toolkit Query (RTK Query) simplifies this process by providing built-in caching, automatic request deduplication, and caching management. But what happens when your app scales up, with more endpoints, complex queries, and larger datasets?
In this blog post, we’ll dive into strategies and best practices for optimizing Redux Toolkit Query for large-scale Next.js applications. We’ll explore advanced techniques for managing caching, handling complex queries, and ensuring a maintainable, scalable architecture.
Why Use Redux Toolkit Query in Large-Scale Applications?
RTK Query is an excellent tool for managing API interactions, especially in large-scale applications due to its:
- Automated Caching: Keeps API responses cached and prevents redundant network requests.
- Optimistic Updates: Provides a seamless user experience by updating the UI before the server responds.
- Server-Side Rendering (SSR): Supports SSR in Next.js, making it easy to fetch data both server-side and client-side.
However, as your application grows, it’s important to optimize your implementation of RTK Query to handle increased complexity and ensure scalability.
Step 1: Structuring Your Redux Toolkit Query for Large-Scale Projects
A good structure is the foundation for scalable projects. For large-scale applications, organizing your API services modularly ensures maintainability and flexibility.
Folder Structure Example:
src/
│
├── features/
│ ├── users/
│ │ ├── usersSlice.ts
│ │ ├── usersAPI.ts
│ └── products/
│ ├── productsSlice.ts
│ ├── productsAPI.ts
│
├── services/
│ ├── api.ts
│ ├── users.ts
│ └── products.ts
api.ts
: A shared base for all API configurations (such as base URL).users.ts
andproducts.ts
: Dedicated files for handling separate endpoints and queries.- Slices: Keep your state management and logic for specific domains (e.g., users, products) separate.
Create a Base API Service:
Use RTK Query’s createApi
to define a base API configuration that can be reused across your features.
// src/services/api.ts
import { createApi, fetchBaseQuery } from '@reduxjs/toolkit/query/react';
export const baseApi = createApi({
reducerPath: 'api',
baseQuery: fetchBaseQuery({ baseUrl: '/api' }),
endpoints: () => ({}),
});
Then, create separate service files for each feature:
// src/services/users.ts
import { baseApi } from './api';
export const usersApi = baseApi.injectEndpoints({
endpoints: (builder) => ({
getUsers: builder.query<User[], void>({
query: () => '/users',
}),
}),
});
export const { useGetUsersQuery } = usersApi;
By organizing each feature’s API separately, you ensure the scalability of your project as more features and endpoints are added.
Step 2: Optimizing Caching and Query Invalidations
Caching plays a major role in optimizing performance. RTK Query automatically caches responses and deduplicates requests, but managing cache invalidation effectively in large-scale apps is crucial.
Smart Cache Invalidation
RTK Query provides a flexible system to invalidate queries. In a large app, some resources will need frequent updates (e.g., a list of active users), while others (e.g., configuration settings) might rarely change. Tailor your cache invalidation strategies accordingly.
// src/services/products.ts
import { baseApi } from './api';
export const productsApi = baseApi.injectEndpoints({
endpoints: (builder) => ({
getProducts: builder.query<Product[], void>({
query: () => '/products',
providesTags: (result) =>
result ? result.map(({ id }) => ({ type: 'Products', id })) : ['Products'],
}),
updateProduct: builder.mutation<Product, Partial<Product>>({
query: (product) => ({
url: `/products/${product.id}`,
method: 'PATCH',
body: product,
}),
invalidatesTags: [{ type: 'Products', id: 'LIST' }],
}),
}),
});
export const { useGetProductsQuery, useUpdateProductMutation } = productsApi;
In this example:
providesTags
: Used to track which data is being cached.invalidatesTags
: Ensures that when a product is updated, the product list is refetched.
Customizing Cache Lifetimes
Adjust cache durations based on the nature of the data:
getProducts: builder.query<Product[], void>({
query: () => '/products',
keepUnusedDataFor: 300, // Keep data in cache for 5 minutes
}),
For rarely updated data, you can increase the cache time to avoid unnecessary network requests.
Step 3: Handling Pagination and Infinite Scrolling
In large-scale applications, handling pagination or infinite scrolling is a common requirement. RTK Query makes this process straightforward.
Pagination Example:
// src/services/users.ts
export const usersApi = baseApi.injectEndpoints({
endpoints: (builder) => ({
getUsers: builder.query<PaginatedResponse<User>, number>({
query: (page = 1) => `/users?page=${page}`,
providesTags: ['Users'],
}),
}),
});
export const { useGetUsersQuery } = usersApi;
In your component:
const { data, error, isLoading } = useGetUsersQuery(currentPage);
You can implement infinite scrolling by incrementing currentPage
when the user scrolls to the bottom.
Step 4: Integrating Redux Toolkit Query with Server-Side Rendering (SSR)
Large-scale Next.js applications often require server-side rendering to improve SEO and initial load times. RTK Query supports SSR, but it needs to be configured properly.
Here’s how you can use RTK Query with SSR in Next.js:
Create a getServerSideProps
Function
// pages/index.tsx
import { store } from '../store';
import { productsApi } from '../services/products';
export const getServerSideProps = async () => {
// Prefetch data server-side
await store.dispatch(productsApi.endpoints.getProducts.initiate());
return { props: {} };
};
Make sure that your Redux store is correctly configured to support SSR.
Configure SSR in Your Store
In your Redux store setup (store.ts
):
import { configureStore } from '@reduxjs/toolkit';
import { productsApi } from './services/products';
export const store = configureStore({
reducer: {
[productsApi.reducerPath]: productsApi.reducer,
},
middleware: (getDefaultMiddleware) =>
getDefaultMiddleware().concat(productsApi.middleware),
});
This configuration ensures that API data is pre-fetched during SSR, reducing initial load times and improving SEO.
Step 5: Improving Developer Experience
As your application grows, maintaining and scaling RTK Query services can become challenging. Here are some tips to streamline the development process:
TypeScript Typings for Safer Code
TypeScript can significantly improve the safety and predictability of your codebase, especially in large-scale apps.
interface Product {
id: number;
name: string;
price: number;
}
export const productsApi = baseApi.injectEndpoints({
endpoints: (builder) => ({
getProducts: builder.query<Product[], void>({
query: () => '/products',
}),
}),
});
By strongly typing your queries, you reduce the risk of runtime errors and improve your developer experience.
Efficient Debugging with Redux DevTools
Use Redux DevTools to track your API requests, responses, and state changes. This can help you identify bottlenecks or errors in your API integration.
Conclusion
Optimizing Redux Toolkit Query for large-scale Next.js applications involves managing caching smartly, handling complex queries like pagination and SSR, and ensuring the maintainability of your API services. By following these strategies, you can ensure your app scales efficiently without sacrificing performance.
Next Steps:
- Integrate more advanced caching strategies, such as stale-while-revalidate (SWR).
- Optimize your API handling for different use cases, like real-time updates or heavy data processing.
- Use code-splitting and lazy loading to improve performance in large apps.